We are going to see the Alert Dialog in detail.
First we going to see how to use a normal AlertDialog
The below code is enough to call AlertDialog,
Java
Next we are going to see how to call a AlertDialog with ListView for selection purpose
Java
Next we are going to see how to call a AlertDialog with ListView with Radio button for selection purpose
Java
A sample with all the three examples of alertdialog is follows:
ShowDialog.java
Likewise for multiple choice in the alertdialog we need to use this line
Java
The full source code
Download source
The different types of AlertDialog images will be like this
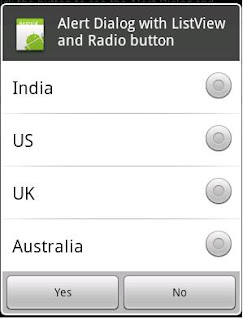
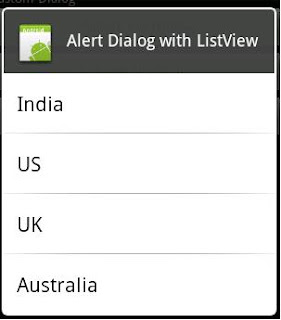
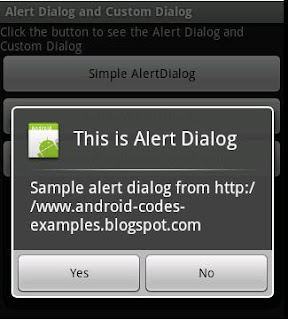
For Custom Dialog you can see this blog http://android-codes-examples.blogspot.com/2011/03/how-to-display-custom-dialog-and.html
Have a good day.
First we going to see how to use a normal AlertDialog
The below code is enough to call AlertDialog,
Java
AlertDialog.Builder alert = new AlertDialog.Builder(ShowDialog.this); alert.setTitle("This is Alert Dialog"); alert.setMessage("Sample alert dialog from http://www.android-codes-examples.blogspot.com"); alert.setIcon(R.drawable.icon); alert.setPositiveButton("Yes", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { Toast.makeText(ShowDialog.this, "Success", Toast.LENGTH_SHORT).show(); } }); alert.setNegativeButton("No", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { Toast.makeText(ShowDialog.this, "Fail", Toast.LENGTH_SHORT).show(); } }); alert.show();
Next we are going to see how to call a AlertDialog with ListView for selection purpose
Java
final CharSequence[] items = {"India", "US", "UK", "Australia"}; AlertDialog.Builder builder = new AlertDialog.Builder(ShowDialog.this); builder.setTitle("Alert Dialog with ListView"); builder.setIcon(R.drawable.icon); builder.setItems(items, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int item) { Toast.makeText(getApplicationContext(), items[item], Toast.LENGTH_SHORT).show(); } }); AlertDialog alert = builder.create(); alert.show();
Next we are going to see how to call a AlertDialog with ListView with Radio button for selection purpose
Java
final CharSequence[] items = {"India", "US", "UK", "Australia"}; AlertDialog.Builder builder = new AlertDialog.Builder(ShowDialog.this); builder.setTitle("Alert Dialog with ListView and Radio button"); builder.setIcon(R.drawable.icon); builder.setSingleChoiceItems(items, -1, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int item) { Toast.makeText(getApplicationContext(), items[item], Toast.LENGTH_SHORT).show(); } }); builder.setPositiveButton("Yes", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { Toast.makeText(ShowDialog.this, "Success", Toast.LENGTH_SHORT).show(); } }); builder.setNegativeButton("No", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { Toast.makeText(ShowDialog.this, "Fail", Toast.LENGTH_SHORT).show(); } }); AlertDialog alert = builder.create(); alert.show();
A sample with all the three examples of alertdialog is follows:
ShowDialog.java
package com.display.dialog; import android.app.Activity; import android.app.AlertDialog; import android.content.DialogInterface; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.Toast; public class ShowDialog extends Activity { /** http://www.android-codes-examples.blogspot.com/ */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); Button alert = (Button) findViewById(R.id.alert); Button customList = (Button) findViewById(R.id.customList); Button customListRadio = (Button) findViewById(R.id.customListRadio); alert.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { AlertDialog.Builder alert = new AlertDialog.Builder(ShowDialog.this); alert.setTitle("This is Alert Dialog"); alert .setMessage("Sample alert dialog from http://www.android-codes-examples.blogspot.com"); alert.setIcon(R.drawable.icon); alert.setPositiveButton("Yes", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { Toast.makeText(ShowDialog.this, "Success", Toast.LENGTH_SHORT).show(); } }); alert.setNegativeButton("No", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { Toast.makeText(ShowDialog.this, "Fail", Toast.LENGTH_SHORT).show(); } }); alert.show(); } }); customList.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { final CharSequence[] items = {"India", "US", "UK", "Australia"}; AlertDialog.Builder builder = new AlertDialog.Builder(ShowDialog.this); builder.setTitle("Alert Dialog with ListView"); builder.setIcon(R.drawable.icon); builder.setItems(items, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int item) { Toast.makeText(getApplicationContext(), items[item], Toast.LENGTH_SHORT).show(); } }); AlertDialog alert = builder.create(); alert.show(); } }); customListRadio.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { final CharSequence[] items = {"India", "US", "UK", "Australia"}; AlertDialog.Builder builder = new AlertDialog.Builder(ShowDialog.this); builder.setTitle("Alert Dialog with ListView and Radio button"); builder.setIcon(R.drawable.icon); builder.setSingleChoiceItems(items, -1, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int item) { Toast.makeText(getApplicationContext(), items[item], Toast.LENGTH_SHORT).show(); } }); builder.setPositiveButton("Yes", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { Toast.makeText(ShowDialog.this, "Success", Toast.LENGTH_SHORT).show(); } }); builder.setNegativeButton("No", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { Toast.makeText(ShowDialog.this, "Fail", Toast.LENGTH_SHORT).show(); } }); AlertDialog alert = builder.create(); alert.show(); } }); } }
Likewise for multiple choice in the alertdialog we need to use this line
Java
new DialogInterface.OnMultiChoiceClickListener() { public void onClick(DialogInterface dialog, int whichButton, boolean isChecked) { /** ... */ } });
The full source code
Download source
The different types of AlertDialog images will be like this
For Custom Dialog you can see this blog http://android-codes-examples.blogspot.com/2011/03/how-to-display-custom-dialog-and.html
Have a good day.
Thanks , your article's realy useful
ReplyDeleteThanks for such a nice article.
ReplyDeleteVery Very Helpfull :)
Good post, This Dialog is more complete,look!!
ReplyDeletehttp://thebestandroide.blogspot.com.es/2014/07/android-context-menu-con-imagen-por.html
Exclusive post.Thanks for sharing.
ReplyDeletePopup builder Plugin Free Download
Great job, This content is very very great content, I got really good information from this content and it helps me a lot, I hope it can help many people like me.
ReplyDeleteFree Source Code
Free Source code For Academic
Academic Project Download
Academic Project Free Download
Freelancer In India