This bar will be like this, the name for this bar is seek bar
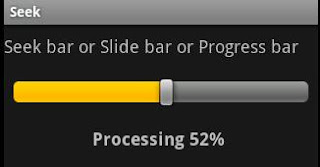
In the xml file we need to mention like this to call the seek bar
xml
The java class file will be like this
Seek.java
The code is fulling checked so you can run the code after downloading it itself.
You can download the full source code
Download source
Have a good day.
In the xml file we need to mention like this to call the seek bar
xml
<SeekBar android:id="@+id/seekbar" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_margin="10px" />
The java class file will be like this
Seek.java
package com.android.seek; import android.app.Activity; import android.media.AudioManager; import android.os.Bundle; import android.widget.SeekBar; import android.widget.TextView; import android.widget.SeekBar.OnSeekBarChangeListener; public class Seek extends Activity { /** Called when the activity is first created. */ AudioManager audioManager; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); final TextView reading = (TextView) findViewById(R.id.reading); SeekBar seekBar = (SeekBar)findViewById(R.id.seekbar); seekBar.setMax(100); seekBar.setOnSeekBarChangeListener(new OnSeekBarChangeListener() { @Override public void onStopTrackingTouch(SeekBar seekBar) { } @Override public void onStartTrackingTouch(SeekBar seekBar) { // TODO Auto-generated method stub } @Override public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) { reading.setText("Processing "+progress+"% "); } }); } }
The code is fulling checked so you can run the code after downloading it itself.
You can download the full source code
Download source
Have a good day.